
Play Store Application link – https://play.google.com/store/apps/details?id=com.ideepro.java25hours
Learn with youtube video-
Quick Overview between Interface, Abstract class and simple class-
Feature | Interface | Abstract Class | Class |
---|---|---|---|
Definition | A set of related methods with empty bodies | A class with one or more abstract methods | A concrete class with a complete implementation |
Syntax | interface | abstract class | class |
Extends another interface | Yes | No | No |
Implements another interface | Yes | Yes | Yes |
Extends another class | No | Yes | Yes |
Can be instantiated | No | No | Yes |
Can have abstract methods | Yes | Yes | No |
Can have concrete methods | No | Yes | Yes |
Can have instance variables | Yes (since Java 8) | Yes | Yes |
All methods are automatically abstract | Yes | No | No |
💡Definition-
Interface is a 100% abstract class.
– Till JAVA 7 – All methods in interface are public and abstract.
In JAVA 8 – public default and public static methods are allowed in java
In JAVA 9 – private methods and private static are allowed in java
-All data members in interface are public, static and final.
– multiple inheritance is allowed in interfaces.
Note 1- class ‘implements’ interface
Note 2- interface ‘extends’ interface
Code samples are here
💡1- One class can implements multiple interfaces
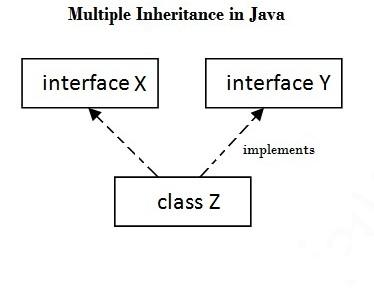
A-
package intrfc; public interface X { void show(); }
B-
package intrfc; public interface Y { void display(); }
C-
package intrfc; public class Z implements X,Y { @Override public void display() { System.out.println("bye"); } @Override public void show() { System.out.println("hi"); } public static void main(String[] args) { Z z=new Z(); z.show(); z.display(); } }
💡2- Interface can extend other interface
A-
package intrfc; public interface X1 { void show(); }
B-
package intrfc; public interface Y1 extends X1 { void display(); }
C-
package intrfc; public class Z1 implements Y1 { @Override public void display() { System.out.println("bye"); } @Override public void show() { System.out.println("hi"); } public static void main(String[] args) { Z1 z=new Z1(); z.show(); z.display(); } }
After Java 7 –
💡1- Interface’s default methods –
public interface Person{ default String getName(){ return “kuldeep kaushik”; } }
Rules of interface’s default methods-
- If the class already has the same method as an Interface, then the default method from the implemented Interface does not take effect.
- If one interface inherits another interface and both of them implement a default method, the default method of the child interface would be used by an implementing class.
- An explicit call to an interface default method can be made from inside an implementing class using super. For example Interface.super.defaultMethod()
💡– Interface’s static methods –
public interface Test{ static String getStaticNumber(){ return 1; } }
Overview comparison of interface methods in Java:
Method | Description |
---|---|
Interface method | An interface method is a method that is declared in an interface and does not have an implementation. |
Interface static method | An interface static method is a static method that is declared in an interface and does not have an implementation. It must be implemented by the implementing class. |
Interface private method | An interface private method is not allowed in Java. An interface can only contain public or default methods. |
Interface default method | An interface default method is a method that is declared in an interface and has a default implementation. It can be overridden by the implementing class if needed. |
Interview Questions —
- What is difference between abstract class and interface?
- What is difference between default methods and static methods?