
Play Store Application link – Java to AngularJS in 16 Steps – App on Google Play
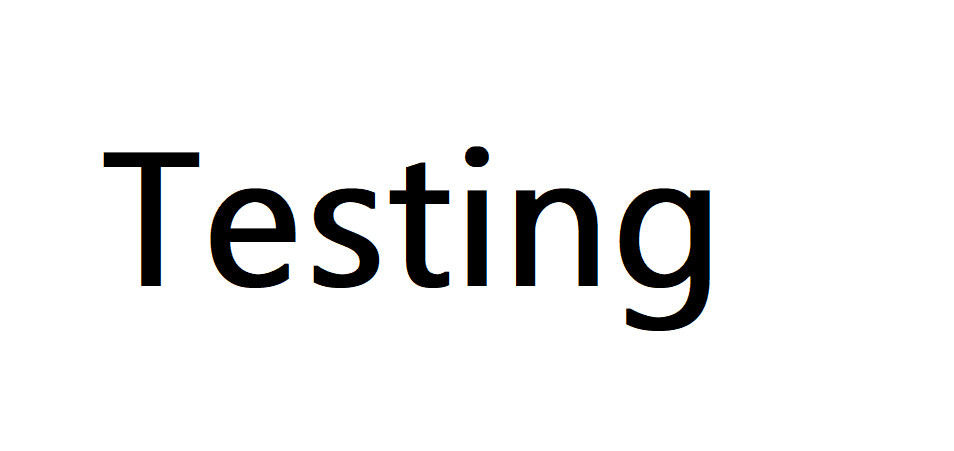
Testing is a crucial part of software development, and AngularJS offers tools to make it easier. As a Java developer, you’re likely familiar with JUnit for unit testing and might have heard of tools like Selenium for end-to-end testing. In AngularJS, similar concepts exist using Jasmine for unit testing and Protractor for end-to-end testing, with Karma serving as the test runner.
Let’s explore testing in AngularJS step by step, while drawing comparisons with familiar Java concepts.
Unit Testing vs End-to-End Testing
Aspect | Unit Testing | End-to-End Testing |
---|---|---|
Scope | Tests individual components in isolation | Tests the entire application (like integration testing) |
Framework | Uses Jasmine | Uses Protractor |
Test Runner | Karma | Protractor |
Dependencies | Uses ngMock to mock services | Uses WebDriver to simulate user interactions |
Java Comparison
In Java, unit testing is often done using JUnit where you test individual methods or classes. In AngularJS, you use Jasmine for the same purpose. Similarly, Selenium is often used for testing the whole web application (end-to-end), which is comparable to using Protractor in AngularJS.
Unit Testing in AngularJS (Using Jasmine)
Unit testing in AngularJS focuses on testing individual components like controllers or services, just like you would test a method in Java using JUnit. In AngularJS, ngMock is used to mock dependencies so you can isolate the part you want to test.
Example: Testing an AngularJS Controller
Here’s how to write a unit test for a controller in AngularJS using Jasmine:
describe('MyController', function() {
var scope, controller;
// Load the module that contains the controller
beforeEach(module('myApp'));
// Inject necessary dependencies
beforeEach(inject(function($rootScope, $controller) {
scope = $rootScope.$new(); // Create a new scope
controller = $controller('MyController', {$scope: scope}); // Instantiate the controller
}));
// Write a simple test case
it('should set greeting to "Hello, World!"', function() {
expect(scope.greeting).toBe('Hello, World!');
});
});
Java Comparison:
In Java, you might write a unit test using JUnit like this:
public class MyControllerTest {
@Test
public void testGreeting() {
MyController controller = new MyController();
assertEquals("Hello, World!", controller.getGreeting());
}
}
In both cases, we are testing a simple behavior of a controller method. In AngularJS, you use scope
to check variables in the controller, similar to how you would call a method in Java and verify its return value.
End-to-End Testing in AngularJS (Using Protractor)
End-to-end testing checks the entire application flow. In Java, you might use Selenium to simulate user actions like button clicks and form submissions. In AngularJS, Protractor serves this purpose, running the tests in real browsers.
Example: End-to-End Test for an AngularJS Application
describe('My App', function() {
it('should display the correct title', function() {
// Load the app in the browser
browser.get('http://localhost:8080');
// Check the title of the page
expect(browser.getTitle()).toEqual('My App');
});
});
Java Comparison:
In a Java environment, you might use Selenium WebDriver for similar testing:
WebDriver driver = new ChromeDriver();
driver.get("http://localhost:8080");
String title = driver.getTitle();
assertEquals("My App", title);
driver.quit();
Both examples focus on verifying that the application loads correctly and displays the expected title.
How Karma Works with Jasmine
In AngularJS, Karma is used as the test runner. It’s responsible for executing your Jasmine tests in different browsers, similar to how Maven or Gradle would run your JUnit tests in Java. Karma continuously runs your tests whenever there are code changes, providing immediate feedback.
Summary
- Unit Testing: Use Jasmine (like JUnit) with the ngMock module to isolate and test components.
- End-to-End Testing: Use Protractor (like Selenium) to test the application’s behavior in the browser.
- Karma: Acts as the test runner to automate and run tests in various environments.
This testing structure allows AngularJS developers to ensure the correctness of their applications, just as Java developers ensure their code with JUnit and Selenium.
By focusing on writing unit and end-to-end tests, you’ll improve your application’s quality and catch bugs early—making your AngularJS apps as robust as your Java backends!