
Play Store Application link – Java to AngularJS in 16 Steps – App on Google Play
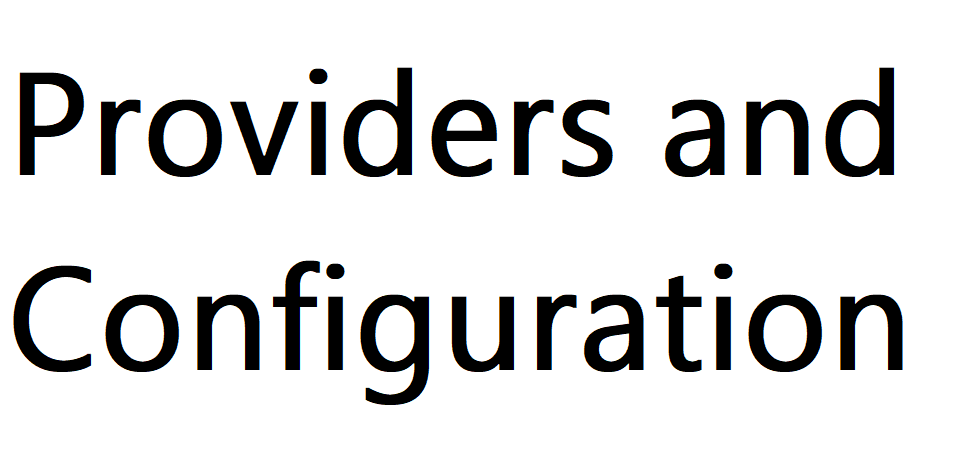
AngularJS Providers and Configuration: A Guide for Java Developers
In AngularJS, providers and configuration are key to setting up and managing services in your application. For Java developers, think of providers and configuration as similar to setting up beans or configurations in a Spring application, but in the context of AngularJS.
Providers in AngularJS
Providers are used to create and configure services in AngularJS. They allow you to set up behavior and inject dependencies before a service is used. There are four main types of providers:
- Constant Provider: Used for defining values that won’t change. This is similar to
public static final
fields in Java. - Value Provider: Used to define values that can be modified. Think of it like a regular class variable in Java, but meant for dependency injection.
- Factory Provider: Defines a service by specifying a function that returns an object. This is similar to a factory method pattern in Java.
- Service Provider: Defines a service using a constructor function, and AngularJS will create an instance of the service using the
new
keyword. This is comparable to defining a bean in Spring where AngularJS handles instance creation.
Java Comparison
- Constant and Value Providers: Comparable to defining constants and variables in Java that are injected where needed.
- Factory Provider: Similar to using factory methods in Java to create instances.
- Service Provider: Equivalent to defining beans or components in frameworks like Spring.
Example Code
Using Factory Provider
Here’s how you can define a service using a factory provider:
angular.module('myApp')
.factory('userService', function() {
var users = [];
function addUser(user) {
users.push(user);
}
function getUsers() {
return users;
}
return {
addUser: addUser,
getUsers: getUsers
};
});
Explanation: This factory provider defines userService
with methods to add and get users, similar to a factory method in Java.
Injecting and Using the Service
angular.module('myApp')
.controller('UserController', function(userService) {
this.users = userService.getUsers();
this.addUser = function(user) {
userService.addUser(user);
};
});
Explanation: The UserController
injects userService
and uses its methods, similar to injecting a bean in Java.
Configuration in AngularJS
Configuration allows you to set up the routing and other application behavior before the app runs. This is similar to configuring routes and beans in Java-based web applications.
Using $routeProvider for Routing
Here’s an example of configuring routes:
angular.module('myApp')
.config(function($routeProvider) {
$routeProvider
.when('/', {
templateUrl: 'home.html',
controller: 'HomeController'
})
.when('/about', {
templateUrl: 'about.html',
controller: 'AboutController'
})
.otherwise({
redirectTo: '/'
});
});
Explanation: This sets up routing similar to how you might configure URL patterns in a Java Servlet-based application.
Summary
AngularJS providers and configuration are crucial for defining and managing services in your application. They allow you to set up reusable services and configure application behavior in a modular way. For Java developers, this is akin to configuring beans and setting up application context in frameworks like Spring, but adapted for web applications with AngularJS.