
Play Store Application link – Advance java – in 15 steps – Apps on Google Play
Learn with our youtube video –
Comparison between Servlet and Filter.
Servlet | Filter |
---|---|
Used for handling requests and generating responses | Used for pre-processing and post-processing requests and responses |
Can handle any type of request | Can only handle requests that match a certain pattern or condition |
Can access and manipulate the entire request and response | Can only access and manipulate specific parts of the request and response |
Filter is used for pre-processing of requests and post-processing of responses.
Filter Api- it’s a part of servlet api, filter interface can be found in javax.servlet package.
Life cycle-
1- init
2- dofilter
3- destroy
Filterchain interface- its used to invoke other filters in the chain.
Example 11- Web app to show pre-processing text before request is handled by servlet and post-processing text after response is generated by servlet.
Project Structure-
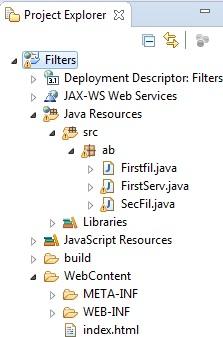
4 files-
1-index.html(inside WebContent)
2-Firstfil.java(inside pacage=’ab’)
3-FirstServ.java(inside pacage=’ab’)
4-SecFil.java(inside pacage=’ab’)
1-index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="ISO-8859-1">
<title>Insert title here</title>
</head>
<body>
<form action="FirstServ">
email
<input type="text" name="e">
password
<input type="password" name="p">
<input type="submit">
<a href="b">View Profile</a>
</body>
</html>
2-Firstfil.java(filter)
package ab;
import java.io.*;
import javax.servlet.*;
@WebFilter("/FirstServ")
public class Firstfil implements Filter {
public void destroy() {
// TODO Auto-generated method stub
}
public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain) throws IOException, ServletException {
String fs=request.getParameter("name");
System.out.println("first filter "+fs);
chain.doFilter(request, response);
System.out.println("after first filter chain-dofilter");
}
public void init(FilterConfig fConfig) throws ServletException {
// TODO Auto-generated method stub
}
}
3-FirstServ.java(servlet)
package ab;
import java.io.*;
import javax.servlet.*;
import javax.servlet.http.*;
@WebServlet("/FirstServ")
public class FirstServ extends HttpServlet {
private static final long serialVersionUID = 1L;
public FirstServ() {
super();
// TODO Auto-generated constructor stub
}
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String fs=request.getParameter("name");
response.getWriter().print(fs);
System.out.println("first servlet "+fs);
// pass the request along the filter chain
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
doGet(request, response);
}
}
4-SecFil.java(filter)
package ab;
import java.io.*;
import javax.servlet.*;
@WebFilter("/FirstServ")
public class SecFil implements Filter {
public void destroy() {
// TODO Auto-generated method stub
}
public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain) throws IOException, ServletException {
// TODO Auto-generated method stub
String fs=request.getParameter("name");
System.out.println("second filter "+fs);
// pass the request along the filter chain
chain.doFilter(request, response);
System.out.println("after second filter chain-dofilter");
}
public void init(FilterConfig fConfig) throws ServletException {
// TODO Auto-generated method stub
}
}