
Play Store Application link – https://play.google.com/store/apps/details?id=com.ideepro.java25hours
Get this in action on Youtube-
Comparison between constructors and methods in Java:
Constructor | Method |
---|---|
Has the same name as the class | Can have any name |
Used to create and initialize an object | Performs a specific task |
Does not have a return type | Can have a return type or be void |
Is called automatically when an object is created | Must be called explicitly |
💡Constructor-
💡Definition-
Constructor is used to construct the objects.
💡-It initialize default or given values to object.
Syntax-
modifiers ClassName(parameters) { //Constructor body }
💡Rules of creation-
1- Constructor Name should be same as class name.
💡Rules of implementation-
1- constructor always should be followed by new keyword.
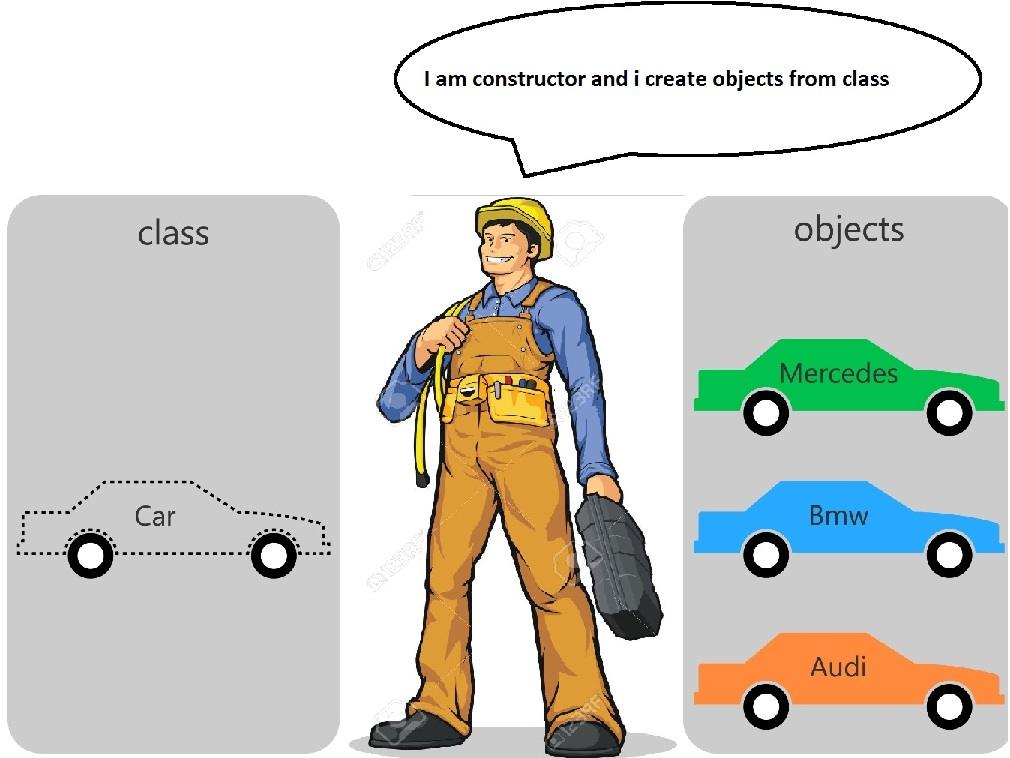
💡Types of constructor-
1- Non Parameterized constructor
2– Parameterized constructor
💡1- Non Parameterized
💡(i)Default constructor-
Only when no constructor is created in class, compiler adds default constructor.
Syntax- created by compiler in compiled code-  constructorName(){ }
💡(ii) Manual Constructor-
Constructor created with no parameters.
It can have statements in constructor body.
Syntax - modifiers constructorName(){ Statements }
💡2- Parameterized Constructor-
Constructor created with parameters.
It also can have statements in constructor body.
Syntax- modifiers constructotName(parameters) { Statements }
Constructor Type | Description |
---|---|
Default Constructor | A default constructor is a constructor that has no parameters. It is provided by the compiler automatically if you don’t write any other constructors for your class. The default constructor initializes the instance variables of the object with their default values (e.g., 0 for integers, false for booleans, and null for reference types). |
Manual Constructor without Parameters | A manual constructor without parameters is a constructor that you define yourself and that has no parameters. You can define a manual constructor without parameters if you want to initialize the instance variables of the object with specific values that are not their default values. |
Parameterized Constructor | A parameterized constructor is a constructor that has one or more parameters. You can define a parameterized constructor if you want to initialize the instance variables of the object with specific values that are passed to the constructor as arguments. |
💡Methods-
method is a set of statements to perform operations
Syntax –
Modifiers returnType methodName(parameters) { //statements }
Note- ReturnType= datatypes + void
code samples are here
1-Program to use default constructor(automatic creation)-
package constructors3; public class Const1 { public static void main(String[] args) { Const1 c1 = new Const1(); } }
2-Program to create manual constructor which prints “a” and then use this manual constructor –
package constructors3; public class Const2 { public Const2() { System.out.println("a"); } public static void main(String[] args) { Const2 c1 = new Const2(); } }
3-Program to create parameterized constructor which takes String s as parameter and then use it by giving “Got it” as argument –
package constructors3; public class Const3 { public Const3(String s) { System.out.println(s); } public static void main(String[] args) { Const3 name = new Const3("Got it"); } }
4-Program to create more than 1 constructor with different parameters then use all constructors by giving arguments.
package constructors3; public class Const4 { public Const4(int i,String a) { System.out.println(i+a); } public Const4(String a,int i) { System.out.println(a+i); } public Const4(double h,char o) { System.out.println(h+" "+o); } public static void main(String[] args) { Const4 c = new Const4(6, "deep"); Const4 c1 = new Const4(65.45d, 'p'); Const4 c2 = new Const4("kaushik",10); } }
Interview Questions —
- What is difference between parameterized and non parameterized constructor?
- What is difference between constructor and method ?