
Play Store Application link – https://play.google.com/store/apps/details?id=com.ideepro.java25hours
Get this in action on youtube-
Feature | Class | Object |
---|---|---|
Definition | A class is a blueprint or prototype for creating objects. | An object is an instance of a class. It is a real-world entity that has state and behavior. |
Syntax | class ClassName { // fields // methods } | ClassName objectName = new ClassName(); |
Example | class Car { int modelYear; String modelName; void startEngine() { … } } | Car myCar = new Car(); |
Properties | A class has properties such as fields and methods. | An object has properties such as its state (the values of its fields) and behavior (the methods it can perform). |
Memory allocation | A class is not physically stored in memory. | An object is physically stored in memory. |
💡Class –
Definitions-
💡 -> Class is a blueprint or template from which objects are created.
💡 -> Class is a logical representation of object.
💡 -> A class can have data members, objects, constructors, methods, nested classes etc
💡 -> Class doesn’t allocated memory when it is created.
💡 -> Class defines data members and methods which objects can implement.
Syntax –
class className{ }
💡Object –
Definitions-
💡-> Object is an instance of a class
💡-> Object is considered as a real world thing.
💡-> Object is a physical entity.
💡-> Object uses data/state and methods/behaviour.
💡-> Object allocates memory when it is created.
Syntax(in general)-
ClassName objectName= new ConstructorName();
Note- ways to create object in java – new keyword(most popular), newInstance() method, clone() method, factory method and deserialization.
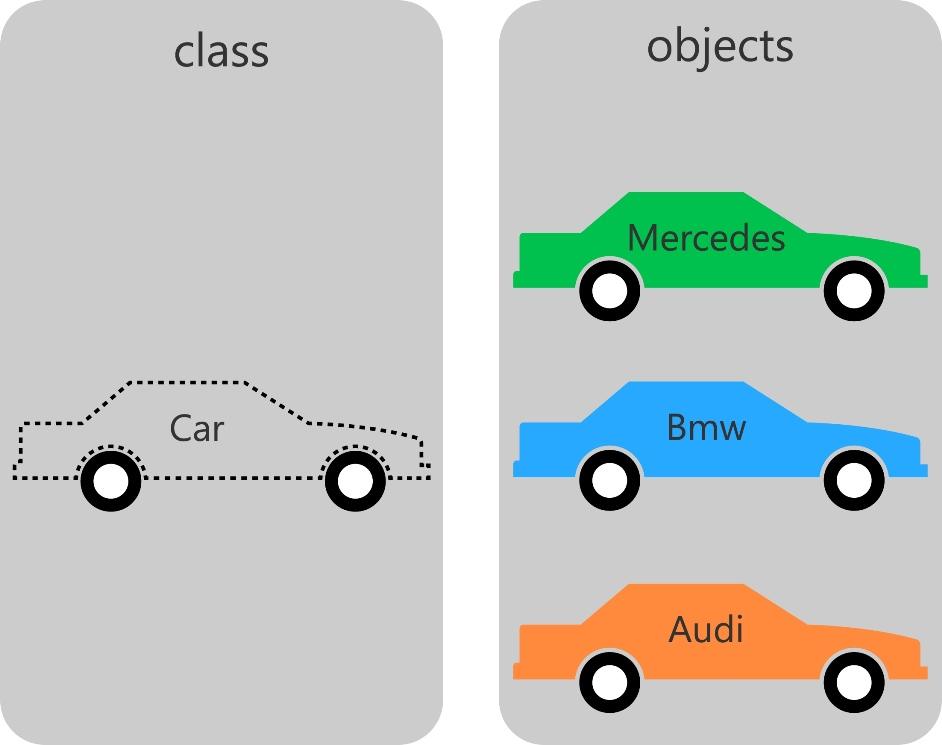
Interview Questions —
- What is class in real world example?
- Can object be created without class?
- Who uses all methods and data defined in any class?