
Play Store Application link – Java to AngularJS in 16 Steps – App on Google Play
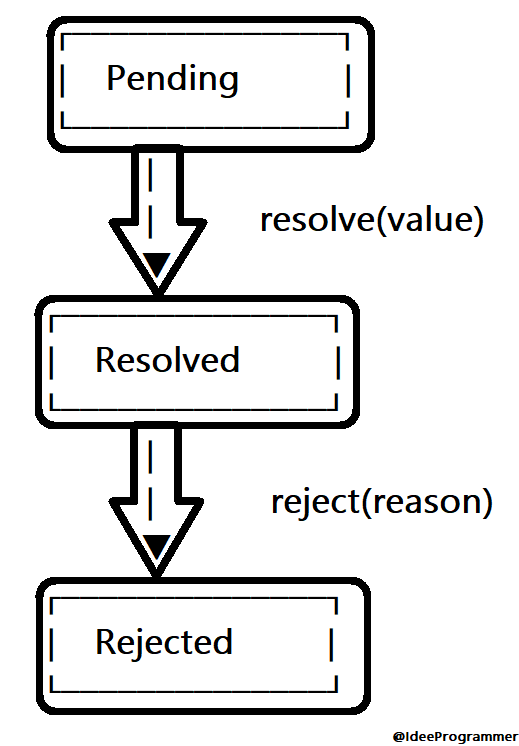
Promises in AngularJS are a way to handle asynchronous operations, such as making HTTP requests, in a cleaner and more manageable way. If you’re familiar with Java, think of Promises as a way to handle tasks that don’t complete immediately, similar to how you might use Future
objects in Java.
Java Comparison:
In Java, you might use Future
or CompletableFuture
to handle asynchronous tasks. Promises in AngularJS serve a similar purpose by representing an operation that will complete in the future.
Overview of Promises in AngularJS
A Promise is an object that represents the eventual outcome of an asynchronous operation. Here’s a quick breakdown of the Promise states:
- Pending: The initial state; the operation is ongoing.
- Resolved: The operation completed successfully.
- Rejected: The operation failed with an error.
Promises help you manage asynchronous code by providing a more structured way to handle results and errors, avoiding deeply nested callbacks.
Creating a Promise
In AngularJS, you use the $q
service to create and manage Promises. The $q
service provides two main methods:
$q.defer()
: Creates a new Deferred object to manually control the Promise’s resolution or rejection.$q.when()
: Creates a Promise that is already resolved with a given value.
Example: Creating a Promise with $q.defer()
Here’s a simple example of creating a Promise that makes an HTTP request:
function getData() {
var deferred = $q.defer(); // Create a new Deferred object
$http.get('/api/data').then(function(response) {
deferred.resolve(response.data); // Resolve the Promise with data
}, function(error) {
deferred.reject(error); // Reject the Promise with an error
});
return deferred.promise; // Return the Promise
}
Java Comparison:
In Java, you might use CompletableFuture.supplyAsync()
to handle asynchronous tasks:
CompletableFuture.supplyAsync(() -> {
// Simulate HTTP request and return data
return "Data";
});
Consuming a Promise
To work with a Promise, you use the then()
method, which takes two functions: one for handling the resolved value and one for handling the rejected error.
Example: Consuming a Promise
getData().then(function(data) {
// Handle the resolved data
console.log("Data received:", data);
}, function(error) {
// Handle the rejected error
console.error("Error occurred:", error);
});
Java Comparison:
Handling results in Java with CompletableFuture
:
CompletableFuture.supplyAsync(() -> {
return "Data";
}).thenAccept(data -> {
// Handle the result
System.out.println("Data received: " + data);
}).exceptionally(ex -> {
// Handle the exception
System.err.println("Error occurred: " + ex.getMessage());
return null;
});
Chaining Promises
You can chain Promises to handle a sequence of asynchronous tasks. Each then()
can return a new Promise, allowing you to handle subsequent tasks.
Example: Chaining Promises
getData().then(function(data) {
return processData(data); // Return a new Promise from processData
}).then(function(result) {
// Handle the result of processData
console.log("Processed data:", result);
}, function(error) {
// Handle the error
console.error("Error occurred:", error);
});
Java Comparison:
Chaining tasks with CompletableFuture
:
CompletableFuture.supplyAsync(() -> {
return "Data";
}).thenApply(data -> {
// Process the data
return "Processed " + data;
}).thenAccept(result -> {
// Handle the processed result
System.out.println("Processed data: " + result);
}).exceptionally(ex -> {
// Handle the exception
System.err.println("Error occurred: " + ex.getMessage());
return null;
});
Summary
Promises in AngularJS are a powerful way to handle asynchronous operations by providing a structured approach to managing results and errors. They help you avoid deeply nested callbacks and make your code more readable and maintainable. This concept is similar to using Future
or CompletableFuture
in Java, allowing you to manage tasks that will complete in the future.