
Play Store Application link β Java to AngularJS in 16 Steps – App on Google Play
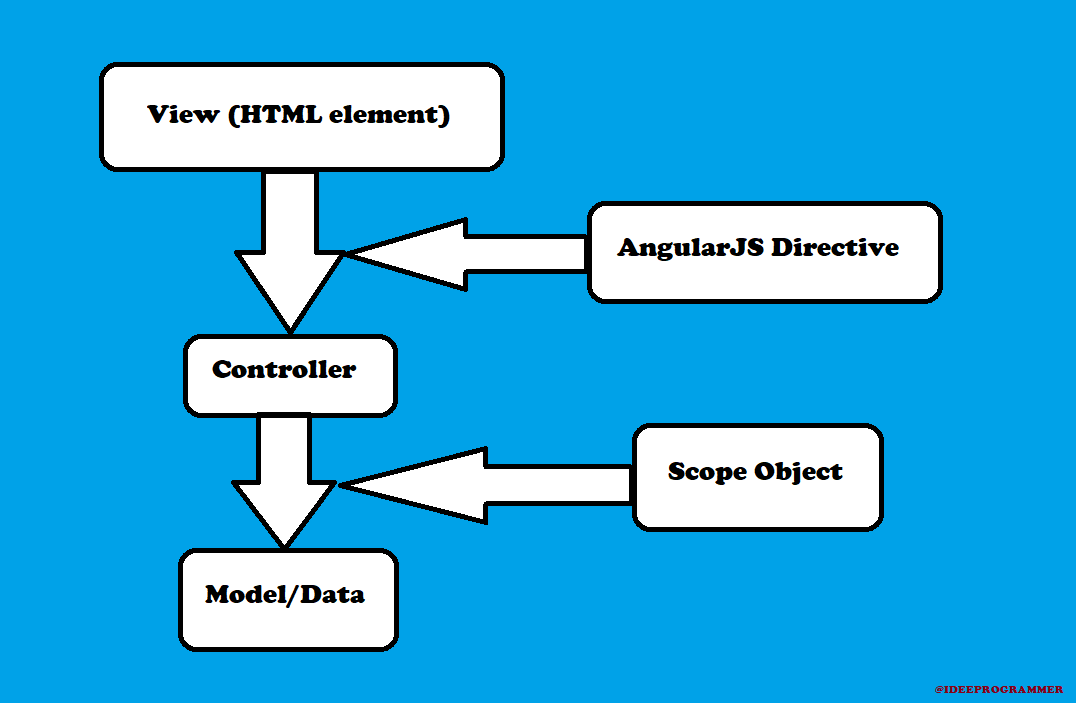
What is Scope in AngularJS?
In AngularJS, scope is an object that binds the HTML view to the controller. It’s used to define properties and functions that can be accessed in both the view (HTML) and the controller (JavaScript). You can think of it as similar to how data is shared between Java classes and JSP pages using Servlets
or Request
objects.
Java Comparison:
In Java, when you pass data from a Servlet
to a JSP, you typically use the HttpServletRequest
or HttpSession
objects to store variables that can be accessed in the JSP. Similarly, AngularJS uses $scope
and $rootScope
to share data between the controller and the view.
Java Example:
// Java Servlet Example
HttpServletRequest request = ...;
request.setAttribute("message", "Hello from Servlet");
AngularJS Equivalent:
// AngularJS Scope Example
$scope.message = "Hello from AngularJS";
Types of Scopes in AngularJS
There are two main types of scopes in AngularJS:
- $scope: This is used for the current view (a specific HTML page). Each controller has its own
$scope
, similar to how each JSP page has its ownHttpServletRequest
. - $rootScope: This is global and shared across all views (entire application). It behaves like a global variable that can be accessed in any controller, similar to the
HttpSession
in Java.
Example Code
Here’s an example to demonstrate the use of $scope
and $rootScope
in AngularJS.
<!DOCTYPE html>
<html ng-app="myApp">
<head>
<meta charset="UTF-8">
<title>AngularJS Scope Example</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script>
</head>
<body ng-controller="myController">
<h1>{{ message }}</h1>
<p>Current view count: {{ count }}</p>
<p>Total count: {{ totalCount }}</p>
<button ng-click="incrementCount()">Increment Current Count</button>
<button ng-click="incrementTotalCount()">Increment Total Count</button>
<script>
var app = angular.module('myApp', []);
app.controller('myController', ['$scope', '$rootScope', function($scope, $rootScope) {
// Local scope variable (specific to this view)
$scope.message = "Welcome to AngularJS Scope Example";
$scope.count = 0;
// Global scope variable (available in all views)
$rootScope.totalCount = 0;
// Function to increment local count (specific to this view)
$scope.incrementCount = function() {
$scope.count++;
};
// Function to increment global count (available to all views)
$scope.incrementTotalCount = function() {
$rootScope.totalCount++;
};
}]);
</script>
</body>
</html>
Explanation:
- $scope: In this example,
$scope.count
is a local variable, which is specific to the current view. This is like how you use theHttpServletRequest
object to store data that is specific to a single HTTP request in a Java web application. - $rootScope:
$rootScope.totalCount
is a global variable accessible across all views. This is similar to how you useHttpSession
to store data across multiple HTTP requests in Java.
Java vs AngularJS Comparison:
- Local Scope ($scope): Like
HttpServletRequest
in Java, used for individual views or requests. Java Example:// Storing data in request scope (specific to a single request) request.setAttribute("count", 0);
AngularJS Example:// Storing data in $scope (specific to this controller/view) $scope.count = 0;
- Global Scope ($rootScope): Similar to
HttpSession
in Java, used for sharing data across multiple views. Java Example:// Storing data in session scope (available across all requests) HttpSession session = request.getSession(); session.setAttribute("totalCount", 0);
AngularJS Example:// Storing data in $rootScope (available across all controllers/views) $rootScope.totalCount = 0;
How It Works:
- $scope.message: The message is displayed in the current view using the
{{ message }}
syntax. - $scope.count: This variable is local to the current controller and is updated every time the “Increment Current Count” button is clicked.
- $rootScope.totalCount: This variable is global and is updated every time the “Increment Total Count” button is clicked. The value is shared across different views and controllers.
Key Takeaways:
- $scope is like
HttpServletRequest
in Java; it’s local to a specific controller or view. - $rootScope is like
HttpSession
; it can be accessed globally across the entire AngularJS application. - You can use these scopes to organize data and functions, similar to how you handle requests and sessions in Java web applications.
By understanding $scope
and $rootScope
, you can easily manage the flow of data between your AngularJS controllers and views, just like handling request and session data in Java.