
Play Store Application link – Java to AngularJS in 16 Steps – App on Google Play
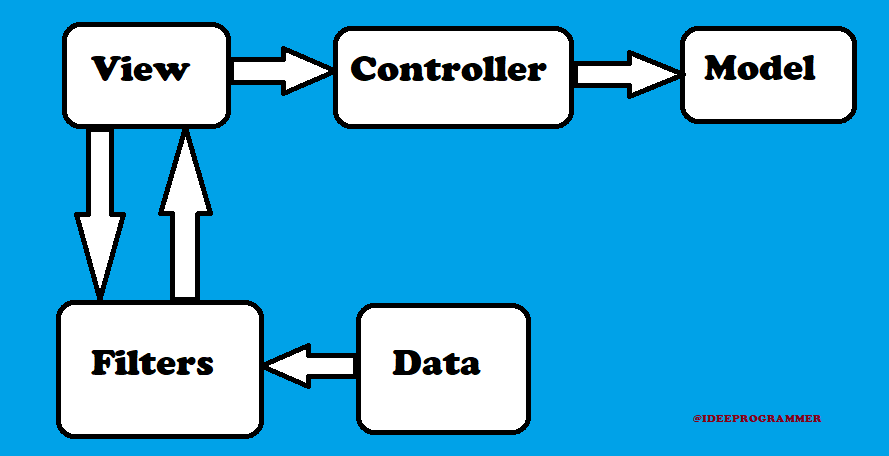
In AngularJS, filters are used to format or transform data before displaying it in the view (HTML). If you’re familiar with formatting or manipulating data in Java, AngularJS filters can be thought of as the equivalent of methods you’d use in Java to format dates, numbers, or strings before sending them to the JSP for display.
Java Comparison:
In Java, you might use utility methods or libraries like SimpleDateFormat
or String.format()
to format data before presenting it in a JSP. Similarly, AngularJS filters allow you to transform data within the HTML itself, directly inside the view.
Java Example (Using SimpleDateFormat):
import java.text.SimpleDateFormat;
import java.util.Date;
public class DateFormatter {
public static void main(String[] args) {
Date today = new Date();
SimpleDateFormat sdf = new SimpleDateFormat("MM/dd/yyyy");
String formattedDate = sdf.format(today);
System.out.println("Formatted Date: " + formattedDate);
}
}
AngularJS Equivalent (Using Filters):
<p>Date: {{date | date:'MM/dd/yyyy'}}</p>
What Are AngularJS Filters?
Filters in AngularJS are used to transform data in the view. These filters allow you to format strings, numbers, dates, and even arrays directly in the HTML, making it much simpler than manually formatting in JavaScript.
Key Types of Filters:
AngularJS comes with a variety of built-in filters. Here are some commonly used ones:
uppercase
: Converts text to uppercase.lowercase
: Converts text to lowercase.currency
: Formats a number as a currency.date
: Formats a date.number
: Formats a number to a specified decimal point.orderBy
: Orders an array based on a property.json
: Formats an object as a JSON string.filter
: Filters an array based on a condition.limitTo
: Limits an array/string to a specified number of items/characters.
Example Code
Below is an example of AngularJS filters. You can save this as an HTML file and open it in a browser.
<!DOCTYPE html>
<html ng-app="myApp">
<head>
<meta charset="utf-8">
<title>AngularJS Filters Example</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script>
<script>
var app = angular.module('myApp', []);
app.controller('myCtrl', function($scope) {
$scope.name = "John";
$scope.amount = 1234.56;
$scope.date = new Date();
$scope.names = [
{name:'John', country:'USA'},
{name:'Mary', country:'Canada'},
{name:'Lucy', country:'Australia'}
];
});
</script>
</head>
<body ng-controller="myCtrl">
<h2>AngularJS Filters Example</h2>
<!-- Original and Uppercase Filter -->
<p>Original name: {{name}}</p>
<p>Uppercase name: {{name | uppercase}}</p>
<!-- Currency Filter -->
<p>Amount: {{amount | currency}}</p>
<!-- Date Filter -->
<p>Date: {{date | date:'MM/dd/yyyy'}}</p>
<!-- Filter with ng-repeat and orderBy -->
<table>
<thead>
<tr>
<th>Name</th>
<th>Country</th>
</tr>
</thead>
<tbody>
<tr ng-repeat="person in names | orderBy:'name'">
<td>{{person.name | lowercase}}</td>
<td>{{person.country | uppercase}}</td>
</tr>
</tbody>
</table>
</body>
</html>
Breakdown of the Example:
- Uppercase and Lowercase Filters:
- AngularJS:
{{name | uppercase}}
converts the name to uppercase in the view. - Java Equivalent: In Java, you’d use
name.toUpperCase()
to achieve this transformation. Java Example:
String name = "John";
String upperName = name.toUpperCase();
- Currency Filter:
- AngularJS:
{{amount | currency}}
formats the number as a currency (in the browser’s locale). - Java Equivalent: You’d use
NumberFormat.getCurrencyInstance()
to format the amount. Java Example:
import java.text.NumberFormat;
double amount = 1234.56;
String formattedAmount = NumberFormat.getCurrencyInstance().format(amount);
- Date Filter:
- AngularJS:
{{date | date:'MM/dd/yyyy'}}
formats the date using the provided format string. - Java Equivalent: You’d use
SimpleDateFormat
to format the date. Java Example:
SimpleDateFormat sdf = new SimpleDateFormat("MM/dd/yyyy");
String formattedDate = sdf.format(new Date());
- OrderBy Filter:
- AngularJS:
ng-repeat="person in names | orderBy:'name'"
sorts the list of names alphabetically. - Java Equivalent: In Java, you’d use
Collections.sort()
to sort a list of objects by a property. Java Example:
List<Person> people = Arrays.asList(
new Person("John", "USA"),
new Person("Mary", "Canada"),
new Person("Lucy", "Australia")
);
Collections.sort(people, Comparator.comparing(Person::getName));
Advantages of Using Filters in AngularJS:
- Ease of Use: Filters are easy to apply directly in the view, reducing the need to write additional JavaScript code.
- Declarative: Filters are applied in a declarative way using
{{}}
, making the code cleaner and easier to maintain. - Reusable: Filters can be reused throughout the application, much like utility methods in Java.
Key Takeaways:
- Filters in AngularJS allow you to format or transform data directly in the HTML, similar to how you would use formatting methods in Java.
- AngularJS syntax (e.g.,
{{name | uppercase}}
) is the equivalent of Java methods (e.g.,name.toUpperCase()
). - Common filters like
currency
,date
,uppercase
, andorderBy
make it simple to display properly formatted data without extra code.
By relating AngularJS filters to common Java formatting functions, you can quickly adapt to using filters to display your data in a clean, readable format within the HTML views.