
Play Store Application link – Java to AngularJS in 16 Steps – App on Google Play
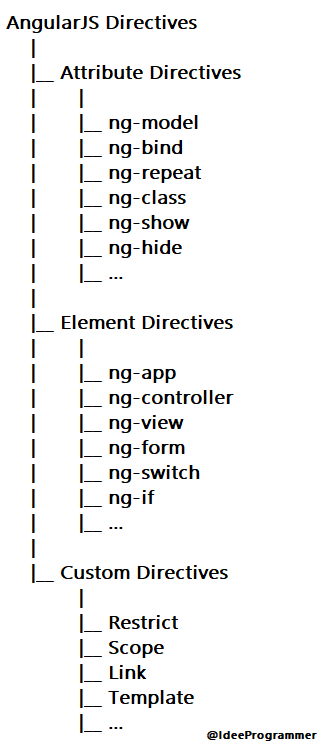
AngularJS Directives introduce AngularJS functionalities to HTML, allowing you to extend HTML attributes and enhance your web applications. For a Java developer familiar with Core Java, Servlets, and HTML, you can think of AngularJS Directives as something similar to how Java annotations work—they add extra behavior to your code without changing the core structure.
#### What is a Directive?
A directive in AngularJS allows you to bind data and add dynamic functionality to HTML elements. In simple terms, it’s like assigning functionality to HTML elements, much like adding attributes or methods in Java.
#### Common Directives
There are 55 built-in directives in AngularJS, but here are the most useful ones:
– `ng-app`: Initializes an AngularJS application, similar to how a `public static void main()` method starts a Java application.
– `ng-init`: Initializes data, like setting initial values in a Java program.
– `ng-model`: Binds the value of an HTML element (such as an input field) to a variable, similar to how you’d define a variable in Java.
– `ng-repeat`: Loops over a collection, much like a `for` loop in Java.
### Java Comparison: Loops and Variables
In Java, you define variables and loop through collections like this:
“`java
String[] names = {“Alice”, “Bob”, “Charlie”};
for (String name : names) {
System.out.println(name);
}
“`
In AngularJS, you use `ng-repeat` to achieve the same looping behavior, and you bind data using `ng-model`. Here’s a simple example:
#### Example 1: Without a Controller
“`html
<!DOCTYPE html>
<html ng-app=””>
<body>
<h1>AngularJS Directives Example</h1>
<div ng-init=”names=[‘Alice’, ‘Bob’, ‘Charlie’]”>
<input type=”text” ng-model=”newName”>
<button ng-click=”names.push(newName)”>Add Name</button>
<ul>
<li ng-repeat=”name in names”>{{name}}</li>
</ul>
</div>
<script src=”https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js”></script>
</body>
</html>
“`
In this example:
– `ng-init` initializes the array `names`, similar to how we initialize an array in Java.
– `ng-model` binds the value of the input field to a variable `newName`.
– `ng-repeat` loops through the `names` array and displays each name in a list.
This example works but isn’t the best practice. In real projects, it’s recommended to define a controller and module, like organizing classes and methods in Java.
#### Example 2: With a Controller (Best Practice)
“`html
<!DOCTYPE html>
<html ng-app=”myApp”>
<body ng-controller=”MyController”>
<h1>AngularJS Directives Example</h1>
<div>
<input type=”text” ng-model=”newName”>
<button ng-click=”addName()”>Add Name</button>
<ul>
<li ng-repeat=”name in names”>{{name}}</li>
</ul>
</div>
<script src=”https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js”></script>
<script>
var app = angular.module(‘myApp’, []);
app.controller(‘MyController’, function($scope) {
$scope.names = [‘Alice’, ‘Bob’, ‘Charlie’];
$scope.addName = function() {
$scope.names.push($scope.newName);
$scope.newName = ”; // Clear the input field after adding
};
});
</script>
</body>
</html>
“`
In this example:
– `ng-app=”myApp”` starts the AngularJS app, similar to how a `main` method starts a Java application.
– `ng-controller=”MyController”` is like defining a class in Java. The controller holds the logic, such as the `addName()` function.
– `$scope.names` is similar to an array in Java. You can add items to it and display them on the webpage.
### Java vs. AngularJS: Method and Scope
In Java, you have class-level variables and methods:
“`java
public class MyController {
private List<String> names = new ArrayList<>(Arrays.asList(“Alice”, “Bob”, “Charlie”));
public void addName(String newName) {
names.add(newName);
}
}
“`
In AngularJS, the `$scope` object holds variables and functions accessible in the HTML view, similar to the `this` keyword in Java.
#### Additional Common Directives
Here’s a list of some other useful directives:
– `ng-click`: Adds a click event handler, similar to attaching an `ActionListener` to a button in Java.
– `ng-hide` / `ng-show`: Toggles visibility based on a condition, like using `if-else` in Java.
– `ng-class`: Dynamically applies CSS classes based on a condition, just like setting attributes conditionally in Java.
For example, using `ng-click` to toggle visibility:
“`html
<div ng-init=”showDetails = false”>
<button ng-click=”showDetails = !showDetails”>Toggle Details</button>
<p ng-show=”showDetails”>These are the details!</p>
</div>
“`
In this case, clicking the button will toggle the display of the paragraph based on the `showDetails` variable. It’s similar to using an `if` condition to show or hide content in Java.
—
This approach allows you to enhance HTML using AngularJS Directives while keeping the logic in JavaScript similar to how you structure your code in Java.
### Key Takeaway
Directives are key to making your HTML dynamic with AngularJS, and they act similarly to how Java annotations or loops work. Use the common directives like `ng-repeat`, `ng-model`, and `ng-click` to bind data and functionality efficiently. The second example, with controllers and modules, is the recommended way to structure your AngularJS code, just like organizing classes and methods in Java.
You can explore all 55 AngularJS directives by referring to the list provided above and experimenting with them in your projects!